The power of symbolic computation meets web design
Tiny web server and hypertext preprocessor built on the freeware wolfram engine with websokets
Get startedWolfram Script Pages
- hypertext preprocessor built on top of Wolfram Kernel -
Embed Mathematica code into HTML/CSS/JS. It works similar to PHP or Mustache template engine.
All graphics objects are converted to SVG strings
<?wsp Table[ ?> <?wsp Graphics3D[i[], ImageSize->Small] ?> <?wsp, {i, {Icosahedron, Octahedron, Tetrahedron}}] ?>
If you need to calculate something more complex, use Module, With, Block as usual. All variables can be global
<ul>
<?wsp
Module[{cities = <||>},
(cities[#[[2, 1]]] = First@Normal@GeoDistance[#, Here]) & /@ GeoNearest[Entity["City"], Here, 5];
With[{max = Max@cities},
cities = #/max & /@ cities;
?>
<?wsp Table[ ?>
<li style="display: block; font-size: <?wsp 1.5 cities[i] ?>em"><?wsp i ?></li>
<?wsp , {i, cities//Keys} ] ?>
<?wsp
]
]
?>
</ul>
List of cities nearby your location scaled by the distance via CSS
- Konigsbrunn
- Augsburg
- Kissing
- Bobingen
- Stadtbergen
The result of the code above
Organize your code into components with designer-friendly HTML templates.
<?wsp LoadPage["page.wsp", {id = "some-id-from-database-or-idunno", other vars...}] ?>
The direct access to the query/session data
<?wsp session ?>
<|Scheme->None,User->None,Domain->None,Port->None,Path->{},Query-><||>,Fragment->None,method->GET,Host->{127.0.0.1},Connection->{keep-alive},Upgrade-Insecure-Requests->{1},User-Agent->{Mozilla/5.0,(Windows,NT,10.0,Win64,x64),AppleWebKit/537.36,(KHTML,,like,Gecko),Chrome/102.0.5005.63,Safari/537.36},Accept->{text/html,application/xhtml+xml,application/xml,q=0.9,image/avif,image/webp,image/apng,*/*,q=0.8,application/signed-exchange,v=b3,q=0.9},Accept-Encoding->{gzip,,deflate},Accept-Language->{ru-RU,ru,q=0.9,en-US,q=0.8,en,q=0.7,de,q=0.6}|>
The result of the code above
Websockets
An easy broadcast to all connected clients from Mathematica
In the real demo the positions of arrow are recalculated on server every second
Every second Mathematica executes the following code
WebSocketBroadcast[server,
With[{
hr = Now[[1, 4]] 180/12 // N,
mn = Now[[1, 5]] 180/60 // N,
sc = Now[[1, 6]] 180/60 // N
},
SetClock[hr, mn, sc]
]
]
On the client side the function SetClock is defined using JS
core.SetClock = function(args, env) {
hr.style = "transform: rotate("+ args[0] +"deg)";
mn.style = "transform: rotate("+ args[1] +"deg)";
sec.style = "transform: rotate("+ args[2] +"deg)";
}
Effortlessly data transfer to across all clients in real-time
Built-in functions in a tiny JS framework allows to use the same syntax as in Mathematica
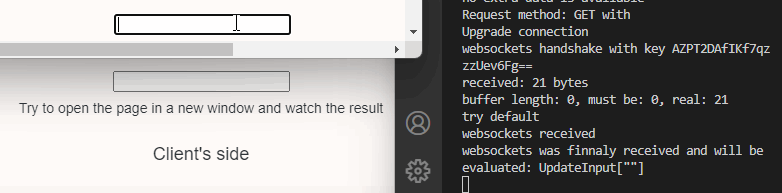
In the real demo text area is mirrored to all clients, and updates on every type. Try to open the page in a new window and watch the result
Client's side
<input id="webinput" type="textarea" value="Type something...">
<script>
const input = document.getElementById('webinput');
input.addEventListener('input', updateValue);
function updateValue(e) {
socket.send(`UpdateInput["${input.value}"]`);
console.log(`${input.value}`);
};
core.SetInput = function(args, env) {
input.value = interpretate(args[0]);
};
</script>
On the Mathematica's side there is only one line
UpdateInput[string_] := WebSocketBroadcast[server, SetInput[string], client]